how to read and write to local Storage using Blazor
first we create a LocalStorageService.cs file
using Microsoft.JSInterop;
using System.Threading.Tasks;
namespace BlazorLocalStorage.Service
{
public class LocalStorageService
{
private readonly IJSRuntime _js;
public LocalStorageService(IJSRuntime js)
{
_js = js;
}
public async Task<string> GetFromLocalStorage(string key)
{
return await _js.InvokeAsync<string>("localStorage.getItem", key);
}
public async Task SetLocalStorage(string key, string value)
{
await _js.InvokeVoidAsync("localStorage.setItem", key, value);
}
}
}
inject the service in Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
services.AddServerSideBlazor();
services.AddScoped<LocalStorageService>();
}
Create a new razor component for testing i called it LocalStorage.razor
@page "/localstorage"
@using BlazorLocalStorage.Service
@inject LocalStorageService Storage
<h1>Testing Local Storage!</h1>
<input type="text" @bind-value="Value" @bind-value:event="oninput" />
<p>Current Value: @Value</p>
<button @onclick="SetValue">Save</button>
<button @onclick="GetValue">Read</button>
@code{
const string key = "blazorKey";
public string Value { get; set; }
async Task SetValue()
{
await Storage.SetLocalStorage(key, Value);
}
async Task GetValue()
{
Value = await Storage.GetFromLocalStorage(key);
}
}
load the app and navigate to /localstorage
type Hello World! and hit save
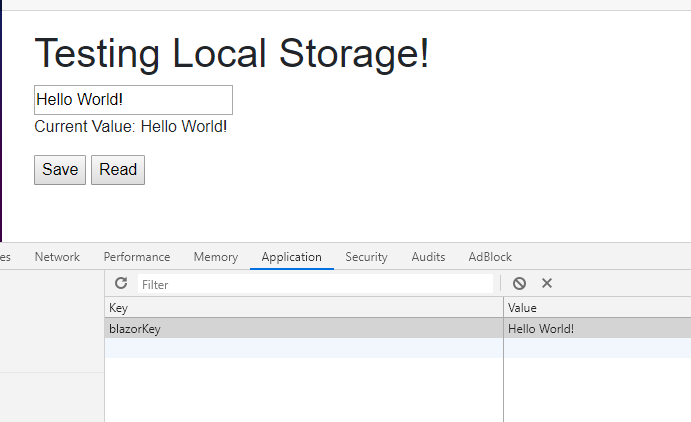
refresh the page a click read to get the message from local storage.