Blazor.tips How to read the browser window dimensions
window.innerHeight and window.innerWidth
first create a service class to read the dimensions from javascript
using Microsoft.JSInterop;
using System.Threading.Tasks;
public class BrowserService
{
private readonly IJSRuntime _js;
public BrowserService(IJSRuntime js)
{
_js = js;
}
public async Task<BrowserDimension> GetDimensions()
{
return await _js.InvokeAsync<BrowserDimension>("getDimensions");
}
}
public class BrowserDimension
{
public int Width { get; set; }
public int Height { get; set; }
}
register the service with the DI on startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
services.AddServerSideBlazor();
services.AddScoped<BrowserService>(); // scoped service
}
add the javascript codes to read the window dimensions you can add them to the _Host.cshtml file directly or add them to a js file
<script type="text/javascript">
window.getDimensions = function() {
return {
width: window.innerWidth,
height: window.innerHeight
};
};
</script>
add new razor file to display the dimensions
@page "/dimensions"
@using BlazorApp.Service
@inject BrowserService Service
<h1>Window Dimensions</h1>
<p>Window Height: @Height</p>
<p>Window Width: @Width</p>
<button @onclick="GetDimensions">Get Dimensions</button>
@code {
public int Height { get; set; }
public int Width { get; set; }
async Task GetDimensions() {
var dimension = await Service.GetDimensions();
Height = dimension.Height;
Width = dimension.Width;
}
}
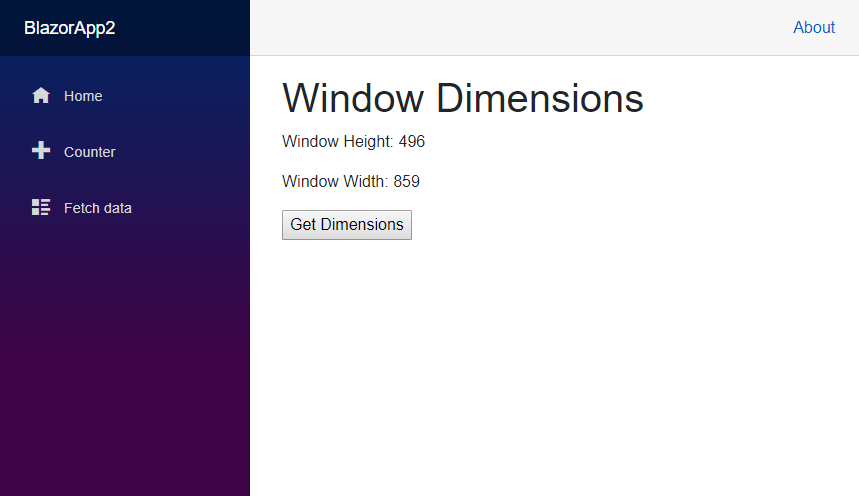
Blazor.tips